How to Check SSL Certificate Expiry for Domains Using Node.js (Nodemailer, TLS)
- Posted on February 15, 2025
- Next/React JS
- By MmantraTech
- 364 Views
Managing SSL certificate expiry dates can be a hassle, especially when handling multiple domains. Missing an expiration date could lead to security issues or website downtime. To simplify this, I’ve built a Node.js utility that automates the process of checking SSL certificate validity across multiple domains.
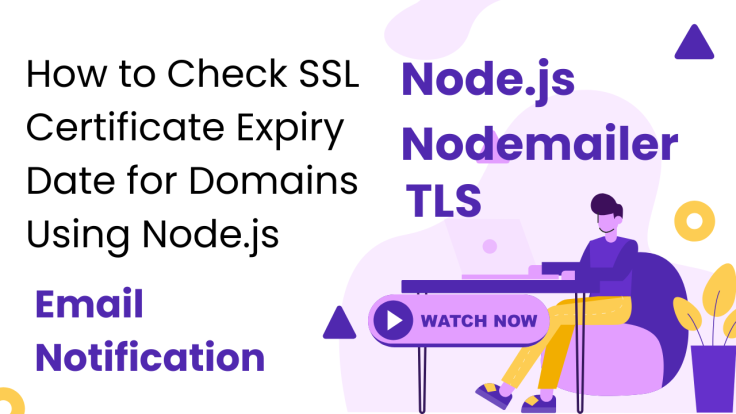
In this blog, I’ll walk you through how to:
✅ Check SSL certificate status for multiple domains using Node.js.
✅ Automate expiry detection to avoid manual checks.
✅ Send email notifications to admins when a certificate expires, using Nodemailer.
By the end of this tutorial, you’ll have a complete solution to monitor SSL certificates and receive alerts before they expire. Let’s dive into the code!
Prerequisites
Before diving into the code, ensure you have:
1️⃣ Node.js installed on your system.
2️⃣ A GoDaddy, Gmail, or any SMTP email account to send alerts.
3️⃣ A list of domains you want to monitor.
Step-by-Step Guide to Implement SSL Expiry Checker in Node.js
1️⃣ Install Required Packages
To begin, create a project directory and initialize a Node.js project:
mkdir ssl-monitor && cd ssl-monitor
npm init -y
Next, install the required dependencies:
npm install nodemailer dotenv
- nodemailer – Sends email notifications.
- dotenv – Loads environment variables securely.
2️⃣ Set Up Environment Variables
Create a .env file to store sensitive credentials:
SMTP_HOST=smtp.your-email-provider.com
SMTP_PORT=465
SMTP_USER=your-email@example.com
SMTP_PASS=your-email-password
NOTIFY_EMAIL=admin@example.com
- Replace SMTP_HOST, SMTP_USER, and SMTP_PASS with your SMTP provider details.
- Set NOTIFY_EMAIL as the email where alerts will be sent.
3️⃣ Write the SSL Expiry Checker Script
3.1 Import Required Modules
const tls = require("tls");
const nodemailer = require("nodemailer");
require("dotenv").config(); // Load environment variables
- tls module allows us to establish a secure connection to check SSL certificates.
- nodemailer sends email alerts.
- .env configuration is loaded for security.
3.2 Define Domains to Monitor
const domains = ["example.com", "yourwebsite.com", "expired.badssl.com"];
let expiredDomains = [];
- Replace the domain names with your list of domains.
- We store expired domains in expiredDomains[].
3.3 Function to Check SSL Expiry
function checkSSLCertificate(domain) {
return new Promise((resolve) => {
const options = { host: domain, port: 443, servername: domain };
const socket = tls.connect(options, () => {
const cert = socket.getPeerCertificate();
if (!cert || !cert.valid_to) {
console.log(`⚠️ Could not retrieve SSL certificate for ${domain}`);
resolve({ domain, expired: true, expiryDate: "Unknown" });
socket.destroy();
return;
}
const expiryDate = new Date(cert.valid_to);
const today = new Date();
const isExpired = expiryDate < today;
socket.end();
resolve({ domain, expiryDate, isExpired });
});
socket.on("error", () => {
resolve({ domain, expired: true, expiryDate: "Unknown" });
});
});
}
✅ What This Function Does:
- Establishes a secure TLS connection to check the SSL certificate.
- Extracts the certificate’s expiration date.
- Returns whether the certificate is expired or valid.
- Handles errors gracefully if the domain has no SSL or cannot be reached.
3.4 Function to Send Email Notifications
async function sendNotification(expiredDomains) {
if (expiredDomains.length === 0) return; // No expired domains, no email needed
const transporter = nodemailer.createTransport({
host: process.env.SMTP_HOST,
port: process.env.SMTP_PORT,
secure: true,
auth: {
user: process.env.SMTP_USER,
pass: process.env.SMTP_PASS,
},
tls: {
minVersion: "TLSv1.2"
},
});
let mailOptions = {
from: process.env.SMTP_USER,
to: process.env.NOTIFY_EMAIL,
subject: "🚨 SSL Certificate Expiry Alert",
text: `The following domains have expired SSL certificates:\n\n${expiredDomains.join("\n")}`,
};
try {
await transporter.sendMail(mailOptions);
console.log("✅ Notification sent successfully!");
} catch (error) {
console.error("❌ Error sending notification:", error);
}
}
✅ What This Function Does:
- Uses Nodemailer to send an email alert to the admin.
- Includes a list of expired domains in the email.
- If there are no expired SSLs, it skips sending an email.
3.5 Check All Domains & Trigger Notifications
async function checkAllDomains() {
console.log("🔍 Checking SSL certificates...\n");
for (let domain of domains) {
const result = await checkSSLCertificate(domain);
console.log(`✅ ${result.domain} - Expiry Date: ${result.expiryDate}`);
if (result.isExpired) {
console.log(`❌ ALERT: SSL Expired for ${result.domain}`);
expiredDomains.push(`${result.domain} (Expired on ${result.expiryDate})`);
}
}
// Send notification if expired SSLs are found
if (expiredDomains.length > 0) {
await sendNotification(expiredDomains);
} else {
console.log("✅ All SSL certificates are valid.");
}
}
// Run the script
checkAllDomains();
✅ What This Function Does:
- Loops through all domains and checks their SSL expiry.
- Logs valid and expired SSL certificates to the console.
- Triggers email notification if any expired certificates are found.
Testing the Script
Run the script using:
node ssl-checker.js
If an SSL is expired, an email notification is sent to the admin.
🎯 Conclusion
With this Node.js SSL monitoring script, you can:
✅ Automate SSL certificate checks across multiple domains.
✅ Prevent website downtime by identifying expired certificates early.
✅ Receive email alerts when SSL certificates expire.
This solution ensures that you never miss an SSL expiry again!
Write a Response