React Js Vite Commands Cheat Sheet
- Posted on July 21, 2024
- Cheat Sheet
- By MmantraTech
- 480 Views
A cheat sheet for common React Vite app commands
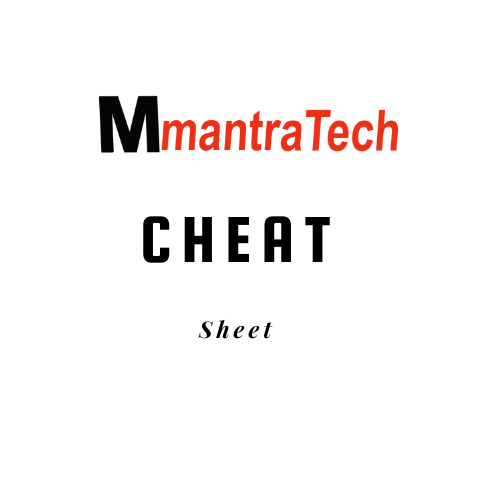
npm create vite@latest my-react-app --template
npm create vite@latest
Project Setup
- Initialize a New Project:
npm init vite@latest my-project-name --template react
yarn create vite my-project-name --template react
pnpm create vite my-project-name --template react
Development
-
Install Dependencies:
npm install
yarn
pnpm install
-
Run Development Server:
npm run dev
yarn dev
pnpm dev
Build and Production
-
Build for Production:
npm run build
yarn build
pnpm build
-
Preview Production Build:
npm run preview
yarn preview
pnpm preview
Scripts (package.json)
{
"scripts": {
"dev": "vite",
"build": "vite build",
"preview": "vite preview"
}
}
Vite Configuration
- Configuration File:
- Vite configuration is typically done in
vite.config.js
orvite.config.ts
.
- Vite configuration is typically done in
Environment Variables
- Define Env Variables:
- Create
.env
,.env.local
,.env.production
, or.env.development
files.
- Create
VITE_API_URL=https://api.example.com
Adding Plugins
- Install Plugins:
- Install the plugin via npm/yarn/pnpm and configure it in
vite.config.js
.
- Install the plugin via npm/yarn/pnpm and configure it in
Example: Adding React Refresh
npm install @vitejs/plugin-react-refresh
// vite.config.js
import reactRefresh from '@vitejs/plugin-react-refresh';
export default {
plugins: [reactRefresh()]
}
Common Dependencies
-
React Router:
npm install react-router-dom
yarn add react-router-dom
pnpm add react-router-dom
-
State Management (e.g., Redux):
npm install @reduxjs/toolkit react-redux
yarn add @reduxjs/toolkit react-redux
pnpm add @reduxjs/toolkit react-redux
Linting and Formatting
-
ESLint:
npm install eslint
yarn add eslint
pnpm add eslint
json{ "scripts": { "lint": "eslint . --ext .js,.jsx,.ts,.tsx" } }
-
Prettier:
npm install prettier
yarn add prettier
pnpm add prettier
Testing
- Testing Library:
npm install @testing-library/react
yarn add @testing-library/react
pnpm add @testing-library/react
Typescript
-
Add TypeScript:
npm install typescript @types/node @types/react @types/react-dom
yarn add typescript @types/node @types/react @types/react-dom
pnpm add typescript @types/node @types/react @types/react-dom
{ "scripts": { "type-check": "tsc --noEmit" } }
Running Scripts
- Run any npm script:
npm run <script-name>
yarn <script-name>
pnpm <script-name>
By following this cheat sheet, you can effectively manage and run your React Vite projects.
Write a Response