How To Install And Use Redux Store In React/Next.js App With Reactjs Toolkit - Practical Tutorial
- Posted on September 7, 2023
- Technology
- By Mmantra Tech
- 676 Views
Prerequisite for Redux Toolkit:
- You must know basics of JavaScript.
- You must know React Js or Next Js or Angular or Vue.
- You must know NODE/NPM/NPX usage.
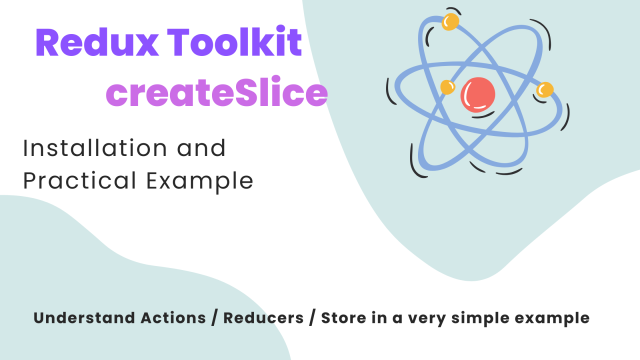
In this tutorial I am goint to take practical example of TODO APP to understand REDUX STORE. We will do below actions.
- Add Task to Store.
- Remove Task from Store.
- Create Next JS App using following command:
- Install redux packages as below
After creating App and installing packagaes you package.json file should look like below:
Your file and folder structure look like the above structure. There may be some difference in your side when you install NEXT Js App 13 Version.
=> You may select "src" folder while installing App or not
You must remember below points if you use Redux either with React Js or Next JS
- All components in React Js by default is "Client Side".
- All Components in Next Js by default is "Server Side" (SSR)
NOTE : Never Ever use "use client" in Server Side Components in NEXT JS
REDUX STORE SETUP in NEXTJS
- Create a folder "redux" at root of the project ( check screenshot above)
- Create a file "Slice.js" in that folder. ( you can choose file name by your own)
Slice.js
"use client"
import { createSlice } from "@reduxjs/toolkit";
const locationSlice = createSlice({
name: "todotask",
initialState: {
todo: []
},
reducers: {
save: (state, param) => {
const { payload } = param;
state.todo = [...state.todo, payload];
},
remove: (state, param) => {
const { payload } = param;
const temp =[...state.todo]
state.todo = temp.filter((item, itemIndex) => {
return itemIndex !== payload
})
},
},
});
const {actions,reducer} = locationSlice
export const {save,remove} = actions;
export default reducer;
Don't worry if you feel above code complicated. If you have basic idead about JavaScript and React Js, I can help you to understand above code. So what is createSlice. According to the official documentation
"A function that accepts an initial state, an object of reducer functions, and a "slice name", and automatically generates action creators and action types that correspond to the reducers and state."
Ok, let's make it very clear by understadning below example. Suppose you have an array in Javascript.
todotasks = [ ] ;
Adding Task
todotasks[0] ="Frist task"
todotasks[1] ="Second task"
todotasks[2] ="Third task"
todotasks[3] ="Fourth task"
Removing Task
todotasks[3] =""
In the above code "todotasks" is a variable state which has initial value is blank array. This is called "Initial State" in createSlice in Redux . When you perform some task such as "Add" and "Remove" then your are performing some Actions . When you define createSlice it is very important to name it because by using name of slice different type of actions are performed and this is the beauty of createSlice ( Redux Toolkit ) that you don't have to create types and constants ( as you used to do before Redux Toolkit ) in different files. Due to Slice of Redux toolkit you can manage Reducers/Actions/Constant in a single file. Reducers are actually pure functins which manage actions in the form of object and they interact with Redux Store to update it.
So in the above code there are two actions managed by reducer.
- save
- remove
and name of the slice is "todotask". When you need to dispatch particular action as per requirement then createSlice automatically mananges Actons type. In our example Auto type will be :
- todotask/save
- toditask/remove
So you must remember when you use createSlice then we have important parameters which are "actions" and "reducer" . In the above example we have destructured our slice and export actions and reducer.
I hope you must have understood basic idea behind createSlice as this article is not about Theory so let's do next step for practical
STEP #3
- Create a file "CustomerProvider.js" inside Redux Folder. and place below code in it.
"use client";
import React from "react";
import { Provider } from "react-redux";
import { store } from "./Store";
const CustomerProvider = ({ children }) => {
return (<Provider store={store}>{children}</Provider>)
};
export default CustomerProvider;
STEP #4
- Create a file "Store.js" inside Redux folder and and place below code in it.
import { configureStore,combineReducers } from "@reduxjs/toolkit";
import todotask from "./Slice";
const rootReducer = combineReducers({
todo: todotask,
//add all your reducers here
},);
export const store = configureStore({
reducer: rootReducer,
});
STEP #5
- Open "layout.js" file (also called special file ) inside Src/App folder and update below code in it
import CustomerProvider from "../../redux/CustomerProvider";
import "./globals.css";
import { Inter } from "next/font/google";
const inter = Inter({ subsets: ["latin"] });
export const metadata = {
title: "Create Next App",
description: "Generated by create next app",
};
export default function RootLayout({ children }) {
return (
<CustomerProvider>
<html>
<body>
{children}
</body>
</html>
</CustomerProvider>
);
}
STEP #6
- Create a new file "AccessStore.jsx" inside src/app Folder and place below code
"use client";
import React, { useState } from "react";
import { useDispatch, useSelector } from "react-redux";
import { save } from "../../redux/Slice";
import { remove } from "../../redux/Slice";
export const AccessStore = () => {
const { todo } = useSelector((state) => state);
const dispatch = useDispatch();
const [task,setTask]=useState("")
const handleTask=(e)=>{
setTask(e.target.value)
}
const handleAdd = () => {
dispatch(save(task));
setTask("")
};
const handleRemove =(index) => {
console.log(index)
dispatch(remove(index));
};
return (
<div className="flex flex-col justify-center items-center mt-10">
{todo.length == 0 ? (
<p>Please Add Task</p>
) : (
todo &&
todo.map((item,index) => {
return (
<div key={index} className="flex justify-between border-2 border-white w-1/2 p-2">
<div className="px-5">{item}</div>
<div className="px-5">
<button
onClick={()=>handleRemove(index)}
class="bg-blue-500 hover:bg-blue-400 text-white font-bold py-2 px-4 border-b-4 border-blue-700 hover:border-blue-500 rounded"
>Remove</button>
</div>
</div>
);
})
)}
<p className="mt-10 flex justify-between items-center w-1/2">
<input type="text" value={task} onChange={handleTask} className="p-2 text-black h-10 mr-2 w-full"/>
<button
onClick={handleAdd}
class="bg-blue-500 hover:bg-blue-400 text-white font-bold py-2 px-4 border-b-4 border-blue-700 hover:border-blue-500 rounded"
>
Add
</button>
</p>
</div>
);
};
STEP #7
- Open "page.js" file (also called special file ) inside Src/App folder and update below code in it
import Image from "next/image";
import { AccessStore } from "./AccessStore";
export default function Home() {
return (
<div>
<AccessStore />
</div>
);
}
That is all you have to do and you are ready to run the ToDo App.
How to run the Next Js App (Dev Env)
By default your App will run in port 3000. You need to copy and past below url .
http://localhost:3000/
and you Browser Screen looks like below screen.
Write a Response