Mastering Middleware In Next.js: Enhancing Web Application Control And Customization.
- Posted on February 8, 2024
- Next.js
- By MmantraTech
- 608 Views
Introduction :
Middleware in Next.js is like a middleman between the incoming request and the server. Imagine you're at a restaurant, and you want to order food. You tell the waiter what you want, and the waiter communicates that to the kitchen. Middleware works similarly, intercepting your request before it reaches the server and performing actions based on it.
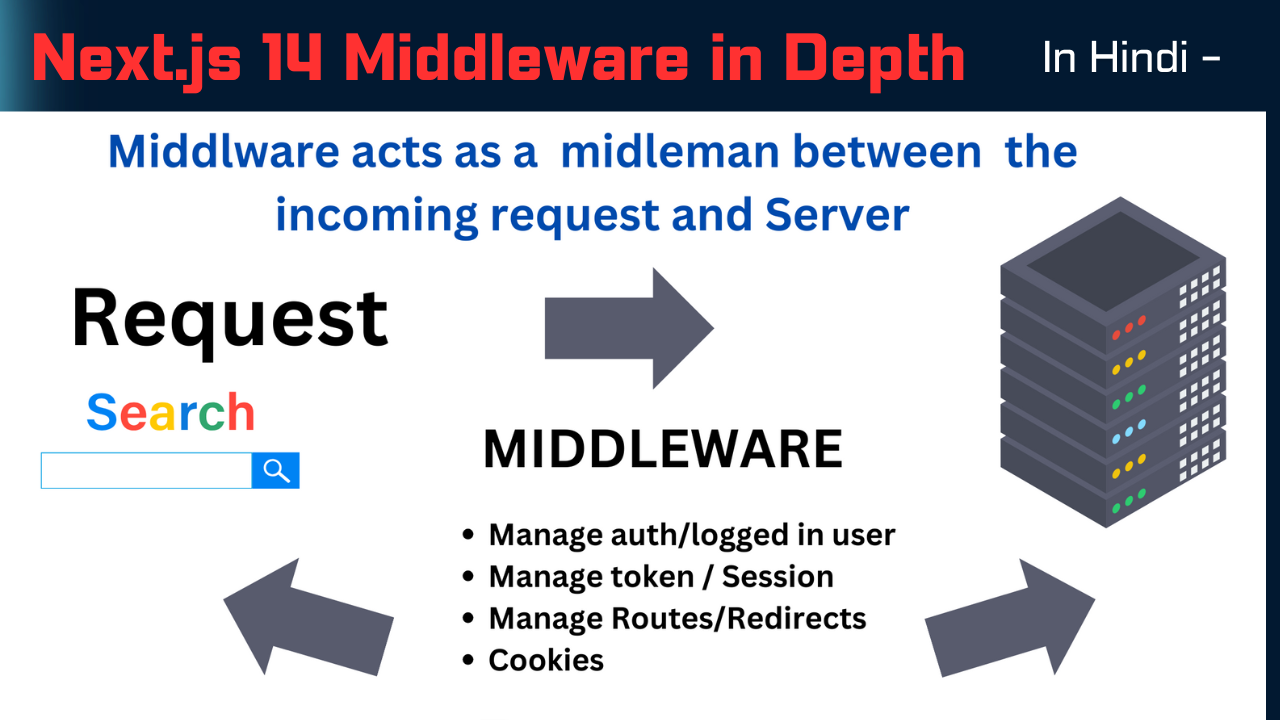
According to the official website :
"Middleware allows you to run code before a request is completed. Then, based on the incoming request, you can modify the response by rewriting, redirecting, modifying the request or response headers, or responding directly."
Let's break down some common aspects of Next.js middleware:
Authentication: Middleware can handle authentication, making sure that only authorized users can access certain pages or perform certain actions on your website. For example, imagine a website where only logged-in users can access their profile page. Middleware can check if the user is logged in before allowing access to that page.
Logging: Middleware can log information about incoming requests, helping developers debug issues or analyze traffic patterns. This can be especially useful for monitoring the performance of your website and identifying any bottlenecks.
import { getToken } from "next-auth/jwt";
export default async function middleware(req) {
// console.log(req.nextUrl)
const pathname = req.nextUrl.pathname;
const { nextUrl } = req;
const isAuth = await getToken({ req,secret:'J59pwqGQE8sD5ow7mVhBIXJ4IHOH8XCLxTqfkMcxpSw=' });
console.log("Isauth>>",isAuth)
const isLoggedIn = !!isAuth;
const isPath = !pathname=="/";
// console.log("auth===",isLoggedIn)
// console.log("pathname===",pathname)
// console.log("isPath===",isPath)
// then you add your logic
// if (pathname.startsWith("/admin/login")) {
// return null;
// }
if(isLoggedIn && pathname.startsWith("/admin/login"))
{
return Response.redirect(new URL("/dashboard", nextUrl));
}
if (pathname!="/" && !isLoggedIn && !pathname.startsWith("/admin/login")) {
console.log("hello")
console.log("hello pathname>>",pathname)
let callbackUrl = nextUrl.pathname;
if (nextUrl.search) {
callbackUrl += nextUrl.search;
}
const encodedCallbackUrl = encodeURIComponent(callbackUrl);
return Response.redirect(new URL(
`/admin/login?callbackUrl=${encodedCallbackUrl}`,
nextUrl
));
// return Response.redirect(new URL("/admin/login", nextUrl));
}
}
export const config = {
matcher: [
// '/dashboard/:path*',
/*
* Match all request paths except for the ones starting with:
* - api (API routes)
* - _next/static (static files)
* - _next/image (image optimization files)
* - favicon.ico (favicon file)
*/
'/((?!api|_next/static|_next/image|images|favicon.ico).*)',
],
}
Authentication by Cookies
import { NextResponse } from "next/server";
import { NextRequest } from "next/server";
import { cookies } from "next/headers";
import { decode } from 'next-auth/jwt';
export default async function middleware(req) {
// const c = req.headers;
// console.log("c===",c)
// if (!request.headers.cookie?.includes('authenticated=true')) {
// }
const authToken = cookies().get("Token")?.value;
const decoded = await decode({
token: authToken,
secret: "J59pwqGQE8sD5ow7mVhBIXJ4IHOH8XCLxTqfkMcxpSw=",
});
console.log("auth===",decoded);
console.log("This is middleware");
}
Error Handling: Middleware can catch errors that occur during the request-response cycle and handle them gracefully. This ensures that your website doesn't crash unexpectedly and provides a better user experience.
Data Parsing: Middleware can parse incoming data from requests, such as form submissions or JSON payloads, making it easier to work with that data in your application.
Response Customization:
Key Features and Enhancements in Next.js 14
The new middleware system in Next.js 14 comes with several improvements over the previous version:
- Global scope: Middleware functions now run at the application level, meaning they apply to all incoming requests, unlike the path-based approach in older versions.
- Simplified API: The new API is more intuitive and easier to use, with a focus on clarity and consistency.
- Conditional execution: You can now define middleware functions that only run under specific conditions, such as on specific paths or for authenticated users.
- Improved performance: The single middleware approach streamlines request processing, potentially leading to better performance compared to nested middleware.
Write a Response