How to Set Up a Node.js Project
- Posted on December 17, 2024
- Technology
- By MmantraTech
- 349 Views
Hi Guys. In this article, I’ll explain the process with simple examples and practical tips to help you how to setup Node.js Project
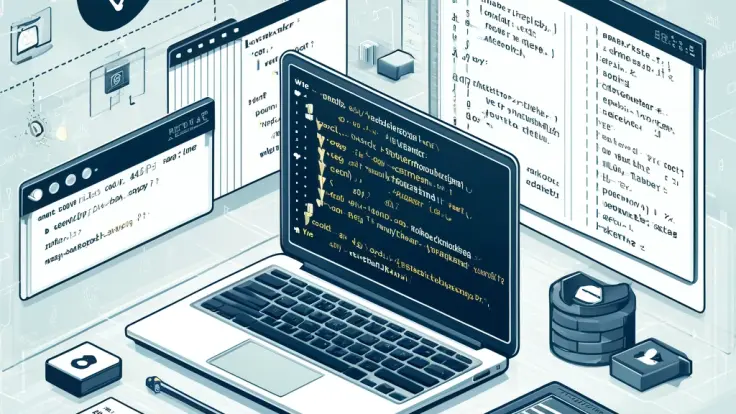
Prerequisites:
1. Node.js Installed: Download and install Node.js from the official website. During installation, the Node Package Manager (npm) is also installed.
2. Code Editor: Use a code editor like Visual Studio Code for better development experience.
3. Basic JavaScript Knowledge: Knowledge of JavaScript syntax is helpful.
Step 1: Create a Project Directory
Start by creating a directory for your project. Open a terminal and run:
mkdir my-nodejs-project
cd my-nodejs-project
Replace my-nodejs-project with your desired project name.
Step 2: Initialize the Project
Run the following command to create a package.json file, which manages your project's metadata and dependencies:
npm init -y
This command initializes the project with default settings. To customize, omit -y and follow the prompts to specify details like project name, version, description, and entry point.
Your directory structure will now include a package.json file:
my-nodejs-project/
package.json
Step 3: Install Dependencies
Node.js uses npm (or yarn) to manage packages. To install a package, use:
npm install <package-name>
For example, to install Express, a popular web framework:
npm install express
To save it as a development dependency, use:
npm install --save-dev <package-name>
This adds the package to the devDependencies section in package.json.
Step 4: Create the Entry Point File
By default, the entry point is index.js. Create this file in the project directory:
touch index.js
Add a simple script to verify your setup:
// index.js
console.log('Hello, Node.js!');
Run the script using:
node index.js
You should see Hello, Node.js! in the terminal.
Step 5: Build a Basic Application
Let’s create a simple HTTP server using Node.js. Replace the contents of index.js with:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, Techmalasi!\n');
});
const PORT = 3008;
server.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}/`);
});
Run the script again:
node index.js
Visit http://localhost:3008 in your browser to see your server in action.
Step 6: Add Scripts to package.json
You can define custom scripts in package.json to simplify commands. Open package.json and add the following under the scripts section:
"scripts": {
"start": "node index.js"
}
Now, you can start your server with:
npm start
Step 7: Use Git for Version Control
Initialize a Git repository to track changes in your project:
git init
git add .
git commit -m "Initial commit"
You can also push the code to a remote repository like GitHub.
Additional Tips
1. Use .gitignore: Create a .gitignore file to exclude node_modules and other unnecessary files from version control.
2. node_modules
3. .env
6. Environment Variables: Use a .env file and the dotenv package to manage environment-specific configurations.
7. Code Linting: Install ESLint for consistent code style:
8. npm install eslint --save-dev
9. npx eslint --init
10. Testing: Add testing frameworks like Jest for automated tests.
Conclusion
So I hope you understand the basic steps of setting up Node.js project. This blog covered creating a project directory, initializing it, installing dependencies, and building a basic application. For more advanced projects, explore tools like TypeScript and PM2 for production deployment.
Visit the official Node.js documentation and the npm documentation for more information.
Write a Response