Most Important Core PHP Interview Questions
- Posted on December 10, 2024
- Technology
- By MmantraTech
- 161 Views
Hi! Are you getting ready for a PHP interview but don’t know where to start? Don’t worry, I’ve got you covered. In this blog, I’ll walk you through some common PHP interview questions, explained step by step in simple terms. Whether you’re just starting out or have some experience, this guide will help you prepare and feel confident. Let’s get started!
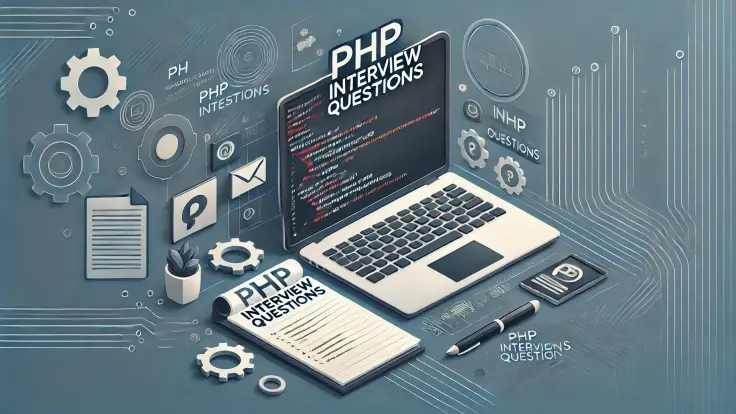
Case 1:
"Find the Maximum Profit from Stock Prices with a Single Buy and Sell"
Problem Description:
You are given an array of integers prices where each element represents the price of a stock on a particular day. Your task is to determine the maximum profit you can achieve by buying and selling the stock exactly once. Note that you must buy the stock before you sell it.
Constraints:
1. The input array prices is non-empty and contains at least two integers.
2. The prices are positive integers.
3. The buy and sell transactions must occur on different days (you cannot sell before you buy).
$prices = [2,6,10,8,9,2,10,70];
Max profit>>68
Explanation:
- Buy at the price 2 (on day 1).
- Sell at the price 70 (on day 8).
- Maximum profit = 70 - 2 = 68.
<?php
$prices = [2,6,10,8,9,2,10,70];
$max_profit=0;
foreach($prices as $key=>$value)
{
if($key < count($prices)-1)
{
$max = max(array_slice($prices,$key+1));
$profit = $max - $value;
if($max_profit < $profit)
{
$max_profit = $profit;
}
}
}
echo "Max profit>>".$max_profit;
Case 2:
"Reposition an Element in an Array to the End of the Array"
Problem Description:
You are given an array arr of integers and an index index. Your task is to move the element at the specified index to the end of the array while preserving the order of the remaining elements.
Input:
1. An array arr containing integers.
2. An integer index specifying the position of the element to be moved.
Output:
An array where the element at the specified index is moved to the end, and all other elements maintain their relative order.
Constraints:
1. The index is valid (i.e., 0 ≤ index < count(arr)).
2. The input array contains at least one element.
$arr = [2, 6, 10, 8, 9, 2, 10, 2, 70];
$index = 4;
$output = [2, 6, 10, 8, 2, 10, 2, 70,9];
<?php
$arr = [2,6,10,8,9,2,10,2,70];
$index=4;
$v = $arr[$index];
unset($arr[$index]);
$arr[]=$v;
print_r(array_values($arr));
Case 3: Find out duplicates in an array and count occurrence of every duplicate element.
Solution 1:
$arr = [2, 6, 10, 8, 9, 2, 10, 2, 70, 6];
// Count occurrences of each value
$counts = array_count_values($arr);
// Print duplicate elements
echo "Duplicate elements are:\n";
foreach ($counts as $key => $value) {
if ($value > 1) {
echo $key . " (repeated $value times)\n";
}
}
?>
Solution 2:
<?php
$arr = [2,6,10,8,9,2,10,2,70,6];
sort($arr);
$final=[];
$prev = $arr[0];
$count=1;
foreach($arr as $key=>$value)
{
if($key<count($arr)-1){
if($arr[$key+1]==$prev)
{
$final[$value]=++$count;
}
else {
$prev=$arr[$key+1];
$count=1;
}
}
}
print_r($final);
Solution 3:
<?php
$arr = [2, 6, 10, 8, 9, 2, 10, 2, 70, 6];
// Initialize an empty array to store occurrences
$occurrences = [];
// Loop through the array
foreach ($arr as $value) {
// Check if the value is already in the occurrences array
if (isset($occurrences[$value])) {
$occurrences[$value]++; // Increment the count
} else {
$occurrences[$value] = 1; // Initialize the count to 1
}
}
// Print the occurrences
foreach ($occurrences as $key => $count) {
echo "Value $key occurs $count times\n";
}
?>
Case 4: You have to reverse of every word in a given string.
<?php
$st="hello world";
$second = explode(" ",$st);
$third=[];
foreach($second as $value){
$third[]= strrev($value);
}
$ans = implode(" ",$third);
print_r($ans);
Case 4: Merger array according to unpacking operator.
<?php
$array1 = [1, 2, 3];
$array2 = [4, 5, 6];
// Use array unpacking (PHP 7.4+)
$combined = [...$array1, ...$array2];
print_r($combined);
?>
Case 5: Insert an element in given array according to index position.
<?php
$arr=[1,2,3,4,5,6];
$inserted =12;
$index=2;
$final = array_splice($arr,$index,0,$inserted);
print_r($final);
print_r($arr);
?>
Array unpacked in php 7.4 +
Add new elememnts using slicing
<?php
// Sample array
$fruits = ['apple', 'banana', 'cherry'];
// New item to insert
$newFruit = 'orange';
// Position to insert (0-based index)
$insertIndex = 1;
// Split the array into two parts at the insert index
$firstPart = array_slice($fruits, 0, $insertIndex);
$secondPart = array_slice($fruits, $insertIndex);
$fruits = [...$firstPart, ...[$newFruit],... $secondPart];
print_r($fruits);
// Insert new item in between the two parts
//$fruits = array_merge($firstPart, [$newFruit], $secondPart);
// Print the modified array
//print_r($fruits);
?>
<?php
$array = array("tech", "techphp", "myntra");
// Insert "orange" at index 1
$newArray = array();
foreach ($array as $key => $value) {
if ($key == 1) {
$newArray[] = "youtube";
}
$newArray[] = $value;
}
$array = $newArray;
print_r($array);
?>
Case 6:
"Group Array Elements by Their First Letter"
Problem Description:
You are given an array of strings where each string represents a name or item. Your task is to group these elements into subarrays based on their first letter, ignoring case. The result should be an associative array where the keys are the lowercase first letters, and the values are arrays containing all the elements that start with that letter.
Input:
An array of strings, e.g.,
$fruits = ['apple', 'banana', 'cherry', 'arvind', 'brijesh'];
Output:
An associative array where:
- Keys are the first letters of the elements (in lowercase).
- Values are arrays of all elements starting with the corresponding letter.
Example Output:
[a] => [ apple, arvind],
[b] => [banana,brijesh],
[c] => [ cherry ]
Explanation:
1. The input array ['apple', 'banana', 'cherry', 'arvind', 'brijesh'] is iterated over element by element.
2. Each element's first letter is extracted and converted to lowercase (e.g., apple → a).
3. If the first letter does not exist as a key in the output array $final_array, a new subarray is created for that letter.
4. The element is added to the subarray corresponding to its first letter.
Constraints:
1. The input array contains only non-empty strings.
2. The grouping is case-insensitive (e.g., Apple and apple are grouped under a).
<?php
// Sample array
$fruits = ['apple', 'banana', 'cherry','arvind','brijesh'];
$final_array=[];
foreach($fruits as $key=>$value)
{
$first_letter = strtolower($value[0]);
if(!isset($final_array[$first_letter]))
{
$final_array[$first_letter]=[];
}
$final_array[$first_letter][]=$value;
}
print_r($final_array);
<?php
// Sample array
$fruits = ['apple', 'banana', 'cherry','arvind','brijesh'];
$final_array= array_flip($fruits);
print_r($final_array);
print_r($final_array['cherry']);
?>
Key Differences Between array_slice() and array_splice():
1. Modification:
o array_slice() does not modify the original array; it creates a new array with the sliced elements.
o array_splice() modifies the original array.
2. Purpose:
o array_slice() is for extracting a portion of an array.
o array_splice() is for removing elements and optionally replacing them.
3. Replacement:
o array_slice() does not support replacement.
o array_splice() allows replacement with new elements.
Write a Response