How to fetch Real time Ticker Data using Fyers Web Socket V3 API in Python
- Posted on January 25, 2025
- Technology
- By MmantraTech
- 153 Views
If you're diving into algorithmic trading and want to connect to the Fyers API to fetch real-time market data, this article will guide you through an example Python implementation. This blog discusses the provided Python script, which utilizes Fyers WebSocket for live data tracking. It explains the code components, highlights its functionality, and provides a human-friendly breakdown of its usage.
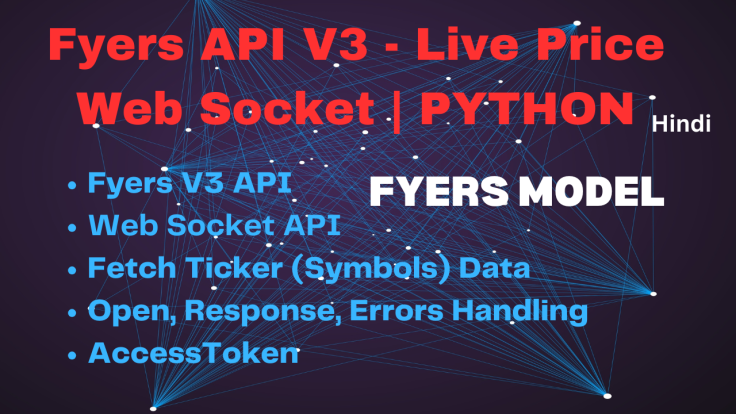
1. Required Libraries and Global Variables
from fyers_apiv3 import fyersModel
import threading
from threading import Thread
from datetime import datetime
from fyers_apiv3.FyersWebsocket import data_ws
fyers_apiv3
: The official Python SDK provided by Fyers to interact with their API.threading
: Used to handle multiple threads for concurrent execution.datetime
: For logging timestamps of incoming messages.
2. The CustomClass
for WebSocket Handling
The main logic for WebSocket connection and real-time data handling is encapsulated in the CustomClass
:
class CustomClass(threading.Thread):
def __init__(self, fyers_ws):
super(CustomClass, self).__init__()
self.fyers_ws = fyers_ws
- Purpose: This class extends
threading.Thread
to allow the WebSocket to run in a separate thread. fyers_ws
: Stores the WebSocket instance.
3. Handling WebSocket Events
The CustomClass
defines several methods to handle WebSocket events:
-
onmessage(self, message):
Triggered when a message is received. It logs the message and extracts the Last Traded Price (LTP) if available.
def onmessage(self, message):
now = datetime.now()
dt_string = now.strftime("%d/%m/%Y %H:%M:%S")
print("Response:", dt_string, ":", message)
if 'ltp' in message:
index = float(message['ltp'])
print(index)
onerror(self, message):
Logs any errors received from the WebSocket.
def onerror(self, message):
print("Error:", message)
onclose(self, message):
Called when the WebSocket connection is closed.
def onclose(self, message):
print("Connection closed:", message)
onopen(self):
Executed when the WebSocket connection is established. It subscribes to specific trading symbols for real-time data.
def onopen(self):
print("Opened>>>>>>>>>>")
symbols = ['NSE:BANKNIFTY25JAN51800CE', 'NSE:BANKNIFTY25JANFUT']
self.fyers_ws.subscribe(symbols=symbols, data_type="SymbolUpdate", channel=15)
self.fyers_ws.keep_running()
4. Connecting to WebSocket
The connect_websocket
method establishes the WebSocket connection using the provided access token:
def connect_websocket(self, access_token):
self.fyers_ws = data_ws.FyersDataSocket(
access_token=access_token,
log_path="",
litemode=False,
write_to_file=True,
reconnect=True,
on_connect=self.onopen,
on_close=self.onclose,
on_error=self.onerror,
on_message=self.onmessage
)
self.fyers_ws.connect()
print("connected>>>", self.fyers_ws.is_connected())
- Access Token: Passed in the format
appid:accesstoken
. - Callback Methods: Attach the methods (
onopen
,onmessage
, etc.) to handle WebSocket events. - Reconnection: Ensures auto-reconnection if the WebSocket disconnects.
5. Running the WebSocket Thread
The run
method starts a new thread for the WebSocket:
def run(self):
access_token = "111EJ0000-100:ILZAQm4EUUYBdGt0rg7F8yCekesLe1C68yKJk1knGcM"
websocket_thread = threading.Thread(target=self.connect_websocket, args=(access_token,))
websocket_thread.start()
- Concurrency: The WebSocket runs in a separate thread, keeping the main thread free for other operations.
6. Instantiating and Running the Thread
t1 = CustomClass(fyers_ws)
t1.start()
-
t1.start()
: Starts theCustomClass
thread, which in turn initiates the WebSocket connection.
The Whole in one Place
from fyers_apiv3 import fyersModel
import threading
from threading import Thread, Event
import time as tm
from fyers_apiv3.FyersWebsocket import data_ws
from datetime import datetime, timedelta
global_threads={}
threads = []
global_threads_queue=dict()
BASE_URL = "https://api-t2.fyers.in/vagator/v2"
BASE_URL_2 = "https://api-t1.fyers.in/api/v3"
URL_SEND_LOGIN_OTP = BASE_URL + "/send_login_otp" # /send_login_otp_v2
URL_VERIFY_TOTP = BASE_URL + "/verify_otp"
URL_VERIFY_PIN = BASE_URL + "/verify_pin"
URL_TOKEN = BASE_URL_2 + "/token"
URL_VALIDATE_AUTH_CODE = BASE_URL_2 + "/validate-authcode"
fyers_ws=''
class CustomClass(threading.Thread):
def __init__(self,fyers_ws):
super(CustomClass, self).__init__()
self.fyers_ws=fyers_ws
def onmessage(self,message):
index=0
now = datetime.now()
dt_string = now.strftime("%d/%m/%Y %H:%M:%S")
print("Response:",str(dt_string),":", message)
if 'ltp' in message:
index=float(message['ltp'])
print(index)
def onerror(self,message):
print("Error:", message)
def onclose(self,message):
print("Connection closed:", message)
def onopen(self):
print("Opened>>>>>>>>>>")
# Specify the data type and symbols you want to subscribe to
data_type = "SymbolUpdate"
# Subscribe to the specified symbols and data type
symbols = ['NSE:BANKNIFTY25JAN51800CE','NSE:BANKNIFTY25JANFUT']
print("symbols>>>",symbols)
self.fyers_ws.subscribe(symbols=symbols, data_type=data_type,channel=15)
# Keep the socket running to receive real-time data
self.fyers_ws.keep_running()
def connect_websocket(self,access_token):
self.fyers_ws = data_ws.FyersDataSocket(
access_token=access_token, # Access token in the format "appid:accesstoken"
log_path="", # Path to save logs. Leave empty to auto-create logs in the current directory.
litemode=False, # Lite mode disabled. Set to True if you want a lite response.
write_to_file=True, # Save response in a log file instead of printing it.
reconnect=True, # Enable auto-reconnection to WebSocket on disconnection.
on_connect=self.onopen, # Callback function to subscribe to data upon connection.
on_close=self.onclose, # Callback function to handle WebSocket connection close events.
on_error=self.onerror, # Callback function to handle WebSocket errors.
on_message=self.onmessage # Callback function to handle incoming messages from the WebSocket.
)
# Establish a connection to the Fyers WebSocket
self.fyers_ws.connect()
print("connected>>>",self.fyers_ws.is_connected())
# if(fyers_ws.is_connected()):
# self.get_live_data()
def run(self):
access_token="111EJ0000-100:ILZAQm4EUUYBdGt0rg7F8yCekesLe1C68yKJk1knGcM"
websocket_thread = threading.Thread(target=self.connect_websocket,args=(access_token,))
websocket_thread.start()
return "hello"
t1=CustomClass(fyers_ws)
t1.start()
Write a Response