How to use array_multisort and array_column in PHP.
- Posted on December 20, 2024
- Technology
- By MmantraTech
- 244 Views
Understanding PHP Array Functions: Sorting Multidimensional Arrays
In this blog post, I will break down a PHP program that demonstrates the use of powerful array functions to sort a multidimensional array. This Blog will explain each function in detail, how they are used, and why they are important.
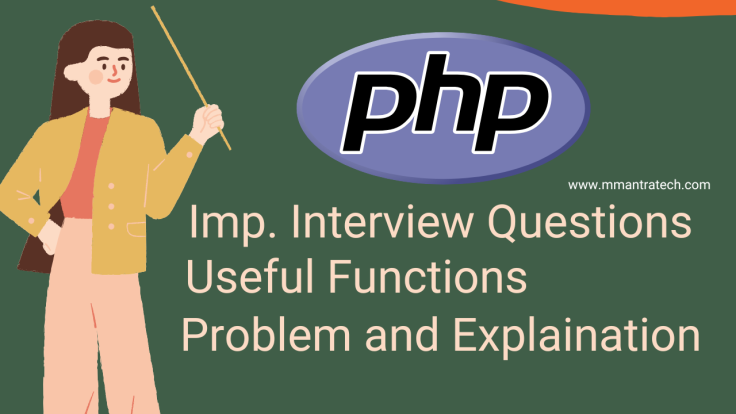
The Program
Here’s the complete code:
$arr = [
["name" => "birbal", "dept" => "HR"],
["name" => "arun", "dept" => "Production"],
["name" => "kamal", "dept" => "HR"],
["name" => "dev", "dept" => "Production"]
];
$name = array_column($arr, 'name');
$dept = array_column($arr, 'dept');
array_multisort($dept, SORT_ASC, $name, SORT_DESC, $arr);
print_r($arr);
Step-by-Step Explanation
1. Initializing the Multidimensional Array
$arr = [
["name" => "birbal", "dept" => "HR"],
["name" => "arun", "dept" => "Production"],
["name" => "kamal", "dept" => "HR"],
["name" => "dev", "dept" => "Production"]
];
This is a multidimensional array where each element is an associative array with two keys:
- name: Represents the name of an employee.
- dept: Represents the department of the employee.
2. Extracting Specific Columns Using array_column()
Syntax:
array_column(array $array, string|int $column_key): array
The array_column() function extracts values from a specific column in a multidimensional array and creates a one-dimensional array with those values.
Code:
$name = array_column($arr, 'name');
$dept = array_column($arr, 'dept');
- $name: Extracts the name column (["birbal", "arun", "kamal", "dev"]).
- $dept: Extracts the dept column (["HR", "Production", "HR", "Production"]).
3. Sorting the Array with array_multisort()
Syntax:
array_multisort(array &$array1, int $sort_order1, ..., array &$arrayN): bool
The array_multisort() function sorts multiple arrays or a multidimensional array based on one or more criteria. It updates the arrays in place and maintains the association between keys.
Code:
array_multisort($dept, SORT_ASC, $name, SORT_DESC, $arr);
Breakdown:
- $dept, SORT_ASC: Sort the dept column in ascending order (e.g., HR comes before Production).
- $name, SORT_DESC: For rows with the same department, sort the name column in descending order.
- $arr: Reorganize the original array ($arr) based on the sorting of $dept and $name.
4. Printing the Sorted Array
Code:
print_r($arr);
The print_r() function prints human-readable information about an array. After sorting, the output will look like this:
Array
(
[0] => Array
(
[name] => kamal
[dept] => HR
)
[1] => Array
(
[name] => birbal
[dept] => HR
)
[2] => Array
(
[name] => dev
[dept] => Production
)
[3] => Array
(
[name] => arun
[dept] => Production
)
)
Key Takeaways
1. array_column(): Simplifies extracting specific columns from a multidimensional array, making the data easier to manipulate.
2. array_multisort(): Enables advanced sorting of arrays by multiple criteria, ideal for structured datasets.
3. Sorting Logic:
o First by dept in ascending order.
o Then by name in descending order.
Practical Applications
- Sorting employee data by departments and names.
- Organizing product inventories by categories and subcategories.
- Simplifying data preparation for APIs and reports.
By understanding and utilizing these PHP functions, you can handle complex sorting scenarios efficiently. Experiment with the program and adapt it to your specific use cases!
Write a Response