PHP Development Complete Course Guide - 2025
- Posted on January 26, 2025
- PHP
- By MmantraTech
- 137 Views
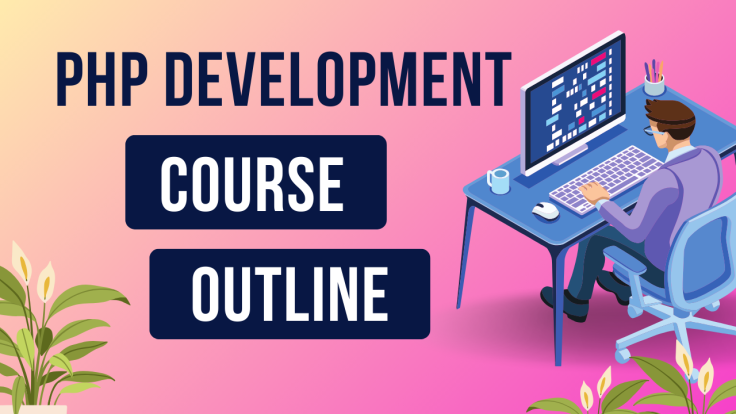
Section 1: Introduction to PHP
-
Overview of PHP
- What is PHP?
- History and evolution of PHP
- Features and capabilities of PHP in 2025
- The role of PHP in modern web development
-
Setting Up the Environment
- Installing PHP 8.3 and configuring it
- Choosing the right IDEs (Visual Studio Code, PhpStorm, etc.)
- Setting up XAMPP, LAMP, or Docker for PHP development
- Introduction to Composer: PHP dependency manager
-
Hello World Program
- Writing your first PHP script
- PHP syntax basics
- Embedding PHP in HTML
Section 2: PHP Basics
-
PHP Syntax and Fundamentals
- Variables and data types
- Constants
- Operators (arithmetic, assignment, comparison, logical, etc.)
- Comments and code readability
-
Control Structures
- Conditional statements: if, else, elseif, switch
- Loops: for, while, do-while, foreach
- Nested control structures
-
Functions in PHP
- Defining and calling functions
- Function parameters and return values
- Variable scope (local, global, static)
- Anonymous functions and arrow functions
-
Error Handling and Debugging
- Types of errors in PHP (syntax, runtime, logical)
- Error reporting and handling with try-catch
- Logging errors and debugging with tools like Xdebug
Section 3: Working with Data in PHP
-
Strings
- String creation, concatenation, and interpolation
- String functions (strlen, substr, strpos, str_replace, etc.)
- Multi-byte string handling with mb_* functions
-
Arrays
- Indexed, associative, and multidimensional arrays
- Array functions (sort, filter, map, reduce, etc.)
- Array destructuring
-
Superglobals and Forms
- Working with $_GET, $_POST, and $_REQUEST
- Handling form submissions
- Input validation and sanitization
-
PHP and Date/Time
- Working with the DateTime class
- Formatting and manipulating dates and times
- Handling timezones
Section 4: Object-Oriented Programming (OOP)
-
OOP Basics
- Classes and objects
- Properties and methods
- Constructor and destructor
-
OOP Principles
- Encapsulation, inheritance, and polymorphism
- Abstract classes and interfaces
- Traits in PHP
-
Advanced OOP
- Namespaces and autoloading
- Static properties and methods
- Magic methods (__construct, __get, __set, __toString, etc.)
- Dependency injection and design patterns (Singleton, Factory, etc.)
Section 5: PHP and Databases
-
Introduction to Databases
- Overview of relational databases
- Setting up MySQL or MariaDB
- Introduction to database tools (phpMyAdmin, Adminer, etc.)
-
Database Connectivity
- Using PDO (PHP Data Objects)
- Executing CRUD operations
- Prepared statements and parameterized queries
-
Advanced Database Handling
- Transactions in PHP
- Working with stored procedures
- Using modern databases like PostgreSQL with PHP
Section 6: Advanced PHP Features
-
File Handling
- Reading and writing files
- Handling file uploads
- Working with file streams
-
Session and Cookies
- Starting and managing sessions
- Setting and retrieving cookies
- Securing session and cookie data
-
PHP and APIs
- Consuming RESTful APIs using cURL and Guzzle
- Creating your own REST APIs with PHP
- Working with JSON and XML
-
PHP Security
- Preventing SQL injection
- Cross-Site Scripting (XSS) protection
- Cross-Site Request Forgery (CSRF) protection
- Password hashing and verification using password_hash and password_verify
Section 7: Frameworks and CMS
-
Introduction to PHP Frameworks
- Why use a framework?
- Overview of popular PHP frameworks (Laravel, Symfony, CodeIgniter, etc.)
-
Getting Started with Laravel
- Installing and setting up Laravel
- MVC architecture
- Routing, controllers, and views
-
WordPress Development
- Setting up a WordPress site
- Creating custom themes
- Developing plugins
Section 8: Testing and Deployment
-
Testing in PHP
- Introduction to PHPUnit
- Writing unit tests and integration tests
- Mocking and testing APIs
-
Deployment
- Preparing your PHP application for production
- Using tools like Git for version control
- Deploying with Docker and CI/CD pipelines
- Monitoring and scaling PHP applications
Section 9: Modern PHP Practices
-
PSR Standards
- PSR-1, PSR-4, and PSR-12 for coding standards
- Understanding dependency injection and service containers
-
Performance Optimization
- Profiling and debugging with tools like Xdebug
- Caching with Redis or Memcached
- Using OPcache for PHP
-
PHP in 2025
- New features in PHP 8.3 and beyond
- PHP’s role in serverless computing
Section 10: Capstone Project
-
Building a Complete PHP Application
- Planning and designing the application
- Using Laravel for back-end development
- Implementing a REST API
- Database integration with MySQL
- Testing and deploying the application
This course structure is designed to provide a comprehensive understanding of PHP development, from basics to advanced concepts, while incorporating the latest updates and practices for 2025.
Write a Response