Understanding the Difference Between Single and Double Quotes in PHP | var_dump() and print() in PHP
- Posted on December 22, 2024
- Technology
- By MmantraTech
- 107 Views
Hello everyone! Welcome to this blog, where I will explore key points in PHP that are particularly useful for interview preparation
o Difference between Single and Double quotes
o Difference between var_dump() and print_r()
When working with PHP, choosing between single quotes (') and double quotes (") for strings can sometimes be confusing. Both serve the same fundamental purpose: to define strings. However, they behave differently in certain scenarios, and understanding these differences can help you write more efficient and readable code.
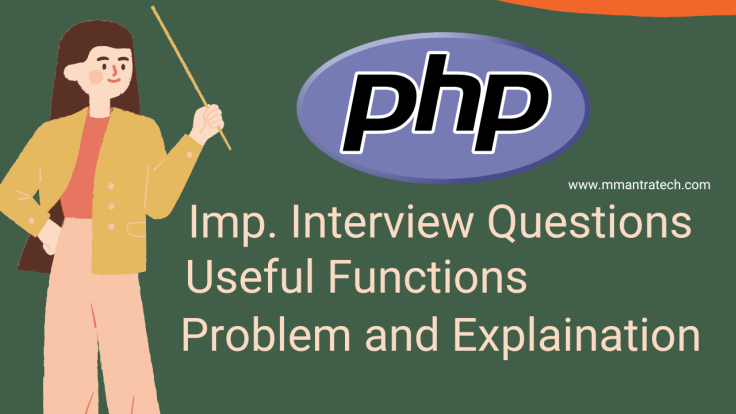
Single Quotes (')
Single quotes are straightforward and treat the content inside them as plain text. They do not process special characters or variables, except for escaping a single quote (\') or a backslash (\\).
Example:
$name = 'techmalasi;
$message = 'Hello, $name';
echo $message; // Output: Hello, $name
Here, $name is treated as plain text because single quotes do not parse variables.
If you need to include a single quote or a backslash within the string, you must escape it:
$text = 'It\'s a beautiful movie!';
echo $text; // Output: It's a beautiful movie!
Double Quotes (")
Double quotes are more versatile because they parse special characters and variables within the string. This makes them ideal for dynamic content.
Example:
$name = "techmalasi";
$message = "Hello, $name";
echo $message; // Output: Hello, techmalasi
In this example, $name is evaluated, and its value is included in the output.
Double quotes also handle escape sequences like \n (newline) and \t (tab):
$message = "youtube,\ntechmalasi!";
echo $message;
Output:
youtube,
techmalasi!
Performance Consideration
Single quotes are slightly faster than double quotes because PHP does not need to evaluate the string for variables or escape sequences. For static strings, using single quotes is a good practice.
Summary Table
Feature |
Single Quotes |
Double Quotes |
Parse Variables |
No |
Yes |
Interpret Escape Sequences |
Limited |
Extensive |
Performance |
Faster |
Slightly Slower |
Understanding var_dump() and print() in PHP
PHP offers multiple ways to output data, and two commonly used functions are var_dump() and print(). Although they may seem similar at first glance, they serve different purposes and are used in distinct scenarios.
var_dump()
The var_dump() function provides detailed information about a variable, including its type and value. This is particularly useful for debugging.
Example:
$number = 42;
$text = "Hello";
$array = [1, 2, 3];
var_dump($number);
var_dump($text);
var_dump($array);
Output:
int(42)
string(5) "Hello"
array(3) {
[0]=>
int(1)
[1]=>
int(2)
[2]=>
int(3)
}
Key Features:
- Displays the type of the variable (e.g., int, string, array).
- Shows the size of the variable (e.g., length of a string or number of elements in an array).
- Useful for complex data structures like arrays and objects.
print()
The print() function is used to output a string. It works similarly to echo, but it has a return value of 1, which makes it slightly less efficient.
Example:
$name = "John";
print("Hello, $name");
Output:
Hello, John
Key Features:
- Outputs simple strings.
- Does not display type or size information.
Difference Between var_dump() and print()
Feature |
var_dump() |
print() |
Purpose |
Debugging complex data |
Outputting strings |
Type Information |
Yes |
No |
Return Value |
None |
1 |
By understanding these differences, you can choose the right tool for your PHP coding needs. Use var_dump() when debugging and inspecting variables, and rely on print() or echo for straightforward output.
Write a Response